SpringBoot整合Swagger
项目完整目录如下
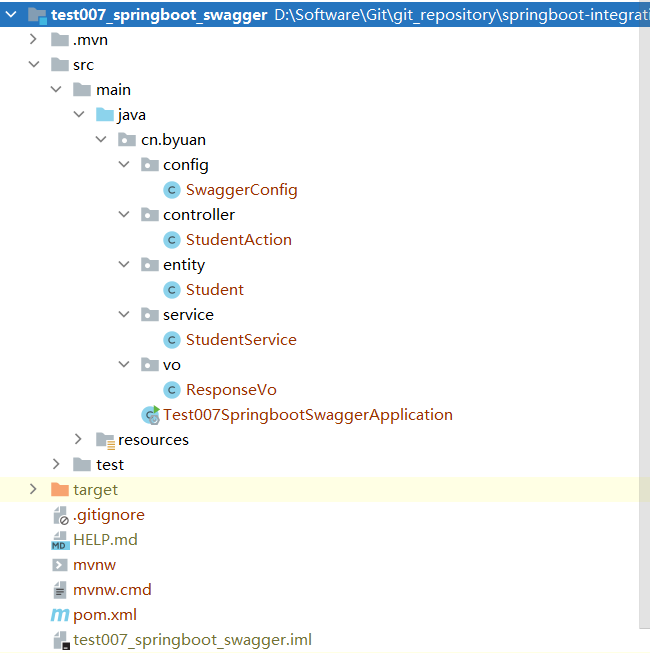
一、创建项目,选择依赖
仅选择Spring Web即可
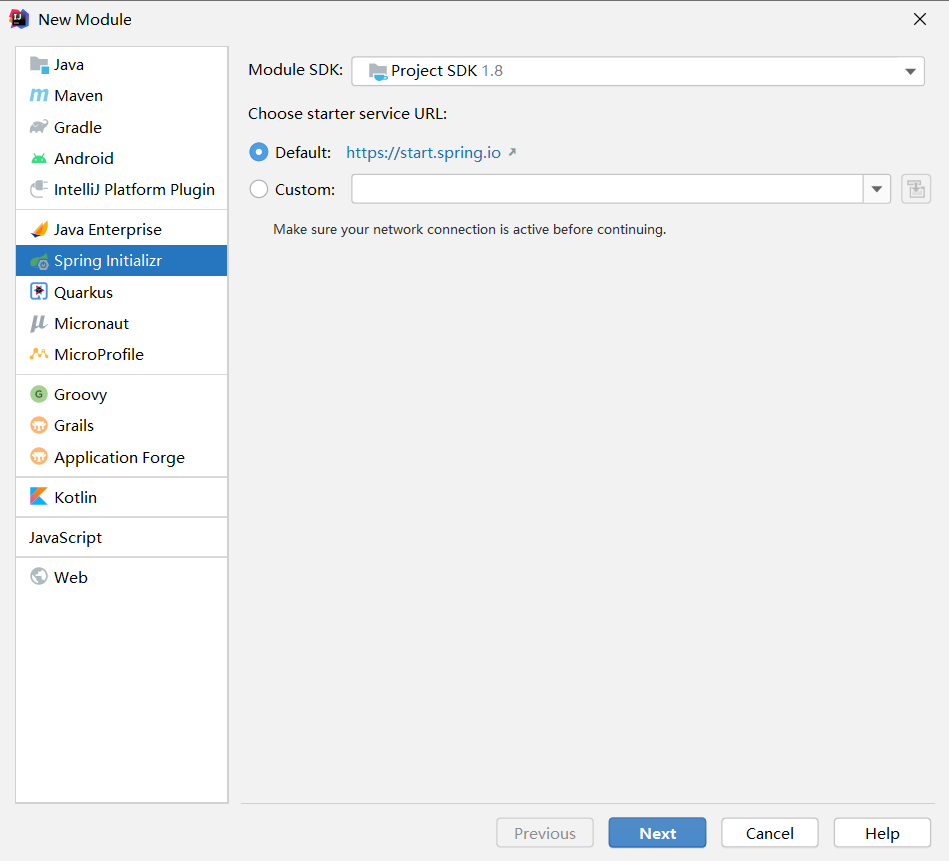
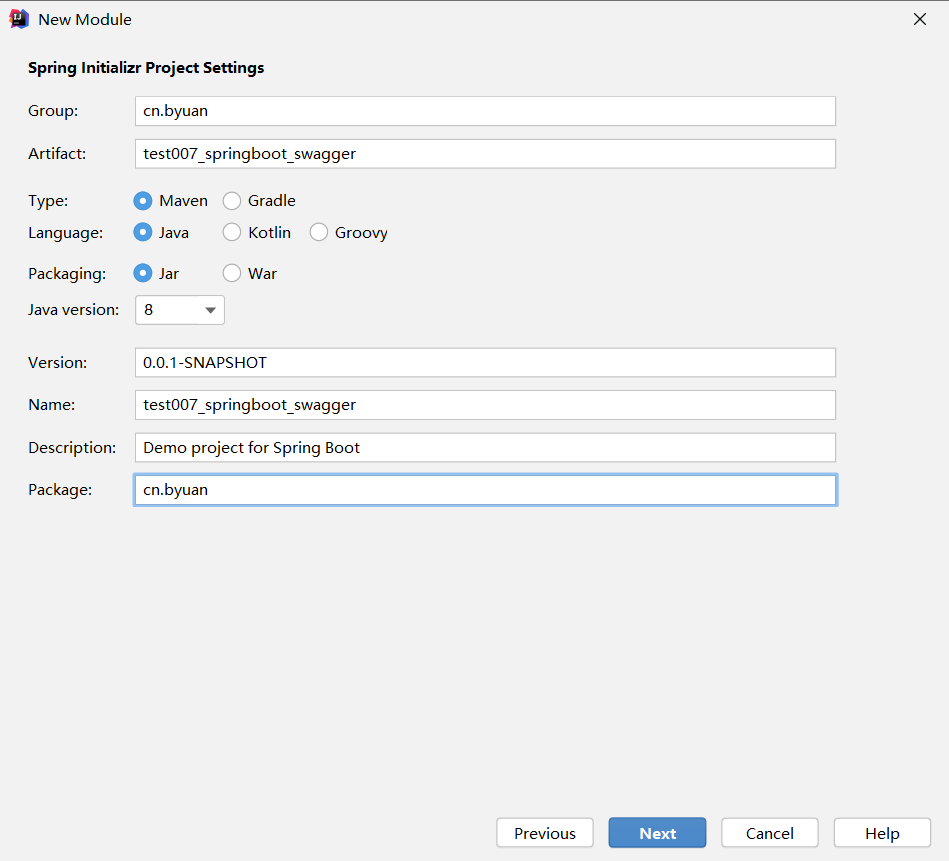
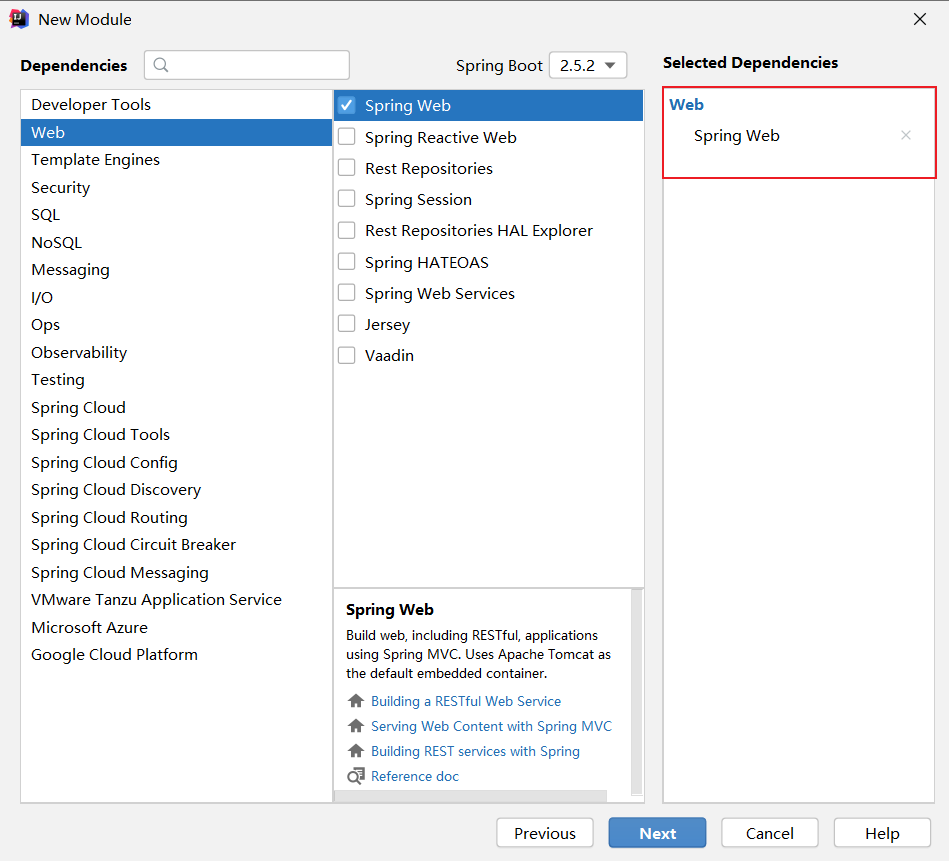
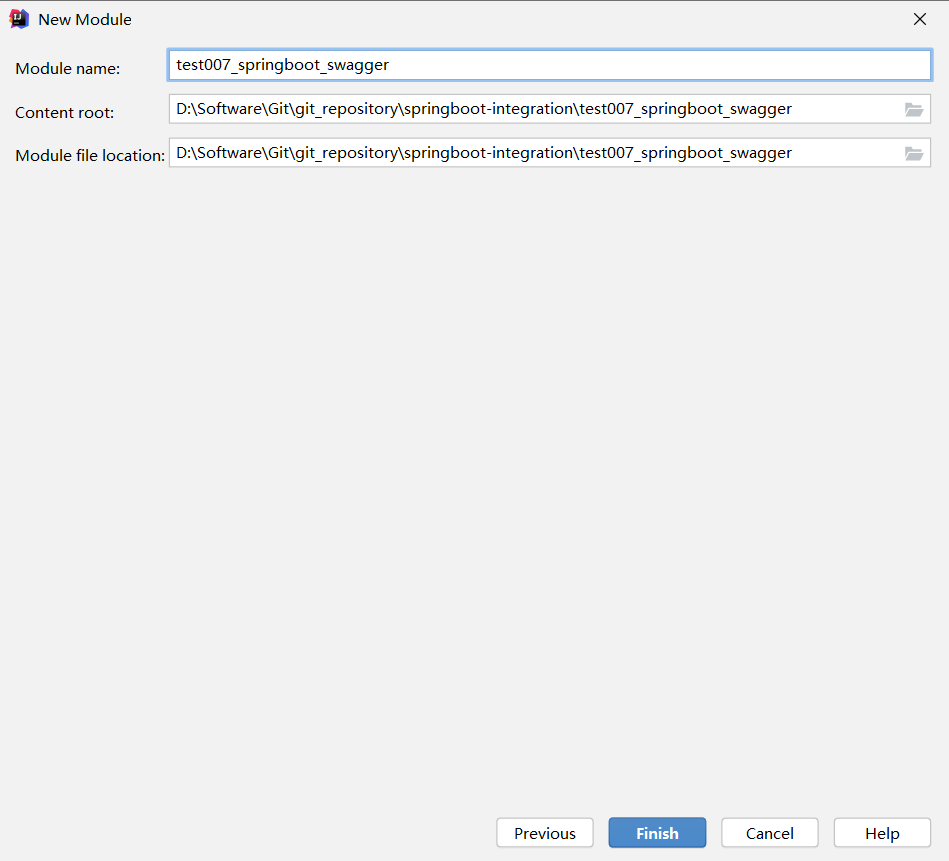
二、在pom文件中引入相关依赖
<!-- 引入lombok --><dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId></dependency><!-- 引入swagger相关的jar --><dependency> <groupId>io.springfox</groupId> <artifactId>springfox-swagger2</artifactId> <version>2.9.2</version></dependency><dependency> <groupId>io.springfox</groupId> <artifactId>springfox-swagger-ui</artifactId> <version>2.9.2</version></dependency>
三、创建Swagger的配置类,并进行配置
package cn.byuan.config;import org.springframework.context.annotation.Bean;import org.springframework.context.annotation.Configuration;import springfox.documentation.builders.ApiInfoBuilder;import springfox.documentation.builders.PathSelectors;import springfox.documentation.builders.RequestHandlerSelectors;import springfox.documentation.service.Contact;import springfox.documentation.spi.DocumentationType;import springfox.documentation.spring.web.plugins.Docket;import springfox.documentation.swagger2.annotations.EnableSwagger2;@Configuration@EnableSwagger2public class SwaggerConfig { @Bean public Docket createRestApi(){ return new Docket(DocumentationType.SWAGGER_2) .pathMapping("/") .select() .apis(RequestHandlerSelectors.basePackage("cn.byuan.controller")) .paths(PathSelectors.any()) .build().apiInfo(new ApiInfoBuilder() .title("SpringBoot整合Swagger") .description("详细信息") .version("1.0") .contact(new Contact( "mtb", "https://www.cnblogs.com/byuan", "byuan98@outlook.com")) .license("The Apache License") .licenseUrl("https://github.com/byuan98/springboot-integration") .build() ); }}
四、发布项目,打开浏览器访问swagger的ui进行测试
五、创建实体类
package cn.byuan.entity;import io.swagger.annotations.ApiModelProperty;import lombok.AllArgsConstructor;import lombok.Data;import lombok.NoArgsConstructor;import lombok.experimental.Accessors;import java.io.Serializable;@NoArgsConstructor// 生成无参的构造方法@AllArgsConstructor// 生成满参的构造方法@Accessors(chain = true)// 使用链式调用@Data// 自动生成get/set方法、重写toString方法等方法public class Student implements Serializable { @ApiModelProperty(value = "学生id")// 对属性进行简要说明 private Integer studentId; @ApiModelProperty(value = "学生姓名") private String studentName; @ApiModelProperty(value = "学生分数") private Double studentScore;}
六、创建vo,对返回结果进行封装
package cn.byuan.vo;import lombok.AllArgsConstructor;import lombok.Data;import lombok.NoArgsConstructor;import lombok.experimental.Accessors;//定义一个返回结果类@NoArgsConstructor@AllArgsConstructor@Data@Accessors(chain = true)public class ResponseVo<E> { private String message; //操作的提示信息 private Integer status; //响应状态码 private E data; //获取数据}
七、创建service层
package cn.byuan.service;import cn.byuan.entity.Student;import cn.byuan.vo.ResponseVo;import org.springframework.stereotype.Service;import java.util.Collection;import java.util.HashMap;import java.util.Map;@Servicepublic class StudentService {// 这里我们不适用数据库, 使用Map集合来模拟数据库中的表 private static Map<Integer, Student> studentMap=new HashMap<>(); private static Integer studentId=10001; static { studentMap.put(studentId, new Student(studentId, "Godfery", 98.5)); studentId++; studentMap.put(studentId, new Student(studentId, "Echo", 95.5)); studentId++; studentMap.put(studentId, new Student(studentId, "Abi", 96.5)); studentId++; }// 插入一名学生返回影响行数 public ResponseVo<Integer> addOneStudent(Student student){ student.setStudentId(studentId); studentMap.put(studentId, student); studentId++; return new ResponseVo<>("插入一条数据成功", 200, 1); }// 删除一位学生返回影响行数 public ResponseVo<Integer> deleteOneStudentByStudentId(Integer studentId){ if(studentMap.containsKey(studentId) == false){ return new ResponseVo<>("您输入的id不存在", 200, 0); } studentMap.remove(studentId); return new ResponseVo<>("删除成功", 200, 1); }// 修改一位学生返回影响行数 public ResponseVo<Integer> updateOneStudent(Student student){ if(studentMap.containsKey(student.getStudentId()) == false){ return new ResponseVo<>("根据学生id,您所修改的学生不存在", 200, 0); } studentMap.put(student.getStudentId(), student); return new ResponseVo<>("学生修改成功", 200, 1); }// 输入studentId查询并返回对应的学生 public ResponseVo<Student> getOneStudentByStudentId(Integer studentId){ if(studentMap.containsKey(studentId) == false){ return new ResponseVo<>("您所查询的学生不存在", 200, null); } return new ResponseVo<>("查询成功", 200, studentMap.get(studentId)); }// 获取所有学生 public ResponseVo<Collection<Student>> getAllStudent(){ return new ResponseVo<>("获取全部学生成功", 200, studentMap.values()); }}
八、创建controller层
package cn.byuan.controller;import cn.byuan.entity.Student;import cn.byuan.service.StudentService;import cn.byuan.vo.ResponseVo;import io.swagger.annotations.Api;import io.swagger.annotations.ApiImplicitParam;import io.swagger.annotations.ApiImplicitParams;import io.swagger.annotations.ApiOperation;import org.springframework.beans.factory.annotation.Autowired;import org.springframework.web.bind.annotation.*;import java.util.Collection;@Api(tags = "学生管理相关接口")@RestController //@Controller + @ResponseBody@RequestMapping("/student"......原文转载:http://www.shaoqun.com/a/859331.html
跨境电商:https://www.ikjzd.com/
黄劲:https://www.ikjzd.com/w/2426
盘古集团:https://www.ikjzd.com/w/1448
飞书互动:https://www.ikjzd.com/w/1319
SpringBoot整合Swagger项目完整目录如下一、创建项目,选择依赖仅选择SpringWeb即可二、在pom文件中引入相关依赖<!--引入lombok--><dependency><groupId>org.projectlombok</groupId><artifactId>lombok</artifactId><
好卖家:https://www.ikjzd.com/w/776
把竞争对手从自己亚马逊Listing页面挤掉,备战2020节日季你不知道的3个广告技巧!:https://www.ikjzd.com/articles/126921
这样打造Listing,第二天他就出了200单!:https://www.ikjzd.com/articles/126920
突发!字节跳动或同意完全放弃TikTok股份:https://www.ikjzd.com/articles/126919
干货:分享7个亚马逊选品小妙招:https://www.ikjzd.com/articles/126918
被两个黑人玩得站不起来了 女朋友被黑人征服小说:http://lady.shaoqun.com/a/247406.html
女友闺蜜好紧好爽再浪一点 女友闺蜜很紧水很多:http://lady.shaoqun.com/a/247865.html
学长每天都在教室要我 二个学长把我弄得好爽:http://lady.shaoqun.com/m/a/248128.html
女海王阵型!不告诉男朋友就勾搭富二代!数百万粉丝,Tik Tok女人,网络名人,做了很多轮:http://lady.shaoqun.com/a/410933.html
清迈的一个天桥小舒林成同志,被居民举报:http://lady.shaoqun.com/a/410934.html
女人为什么选择"暧昧"?倾听中年妇女的心声:http://lady.shaoqun.com/a/410935.html
独立站高昂流量成废品,如何操作才能起死回生?(上):https://www.ikjzd.com/articles/146487